Using Google for geocoding without displaying a map
In a previous blog post I talked about performance tuning SQL queries involving Geographies. In order to use the (now much faster) queries we needed a way of reliably getting a user's approximate location in order to figure out where they were and what the most appropriate content for them is based on that location.
Originally, I thought about using the google.maps.Geocoder but I didn't really want to show a map on our UI - a requirement of using the Geocoder as mentioned in Google's ToS. While looking for alternatives, I stumbled across the places auto complete. It's full of juicy goodness, it's easy to use and the documentation even says:
You can use Place Autocomplete even without a map. If you do show a map, it must be a Google map.
Yay! Here's a screenshot from the documentation:
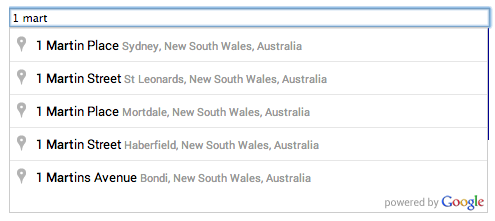
Out of the box it doesn't work exactly as I wanted. I need it to only show results for a certain country and I'm only interested in lat long - I don't care about anything else so I don't want random businesses to get suggested for users. I just passed in some options to achieve the above. Here's some code illustrating it (don't forget to include the places library script https://maps.googleapis.com/maps/api/js?libraries=places&sensor=false)
// Get a reference to the inputvar input = document.getElementById('Location');// Bam! Turn it into a clever Google Autocomplete inputvar autocomplete = new google.maps.places.Autocomplete(input, {types: ['geocode'], // Geocodes onlycomponentRestrictions: {country: 'AU' // Limit me to Australia.}});// Add an event so I know when the user has chosen something.google.maps.event.addListener(autocomplete, 'place_changed', function(e) {// Get the currently selected place.var place = autocomplete.getPlace();// Check that we've actually got a geometry for the resultif (!place.geometry) return;// Do stuff with lat long e.g. place.geometry.location.lat()});
I also created a Google API account and activated the Maps Javascript API.
If you want to build something yourself rather than use the OOTB solution you can use the Places API which is free for 100,000 hits a day (as long as you register).
Thanks Google!
Comments
Post a Comment